Harnessing the Power of WordPress Shortcodes in Your Theme's PHP
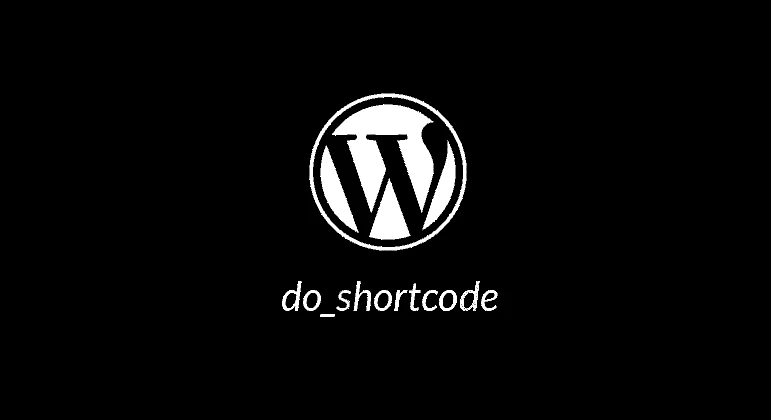
Overview
WordPress shortcodes are powerful tools that allow you to add dynamic content and functionality to your website with minimal effort. While they are commonly used within post content or widgets, integrating shortcodes directly into your theme's PHP files can offer even more flexibility and control over your site's design and functionality. In this guide, we'll explore the ins and outs of using WordPress shortcodes in your theme's PHP files, complete with examples and best practices.
Understanding WordPress Shortcodes
Before diving into integrating shortcodes into your theme's PHP, let's first understand what shortcodes are and how they work. WordPress shortcodes are essentially placeholders that are replaced with dynamic content or functionality when a page or post is rendered. They are enclosed in square brackets, like [shortcode]
, and can accept attributes to customize their behavior.
Why Use Shortcodes in Theme PHP?
While shortcodes are commonly used within post content or widgets, integrating them into your theme's PHP files offers several advantages:
- Enhanced Flexibility: By embedding shortcodes directly into your theme's PHP files, you can customize the layout and functionality of your site beyond what is possible with standard post content or widgets.
- Efficiency: Using shortcodes in theme PHP allows you to reuse complex functionality across multiple pages or templates without duplicating code.
- Consistency: By centralizing shortcode usage within your theme files, you can ensure a consistent user experience throughout your site.
Integrating Shortcodes into Theme PHP
Step 1: Register the Shortcode
Before using a shortcode in your theme's PHP, you need to register it using the add_shortcode()
function. This function takes two parameters: the shortcode name and the callback function that will be executed when the shortcode is encountered.
1function custom_shortcode_function( $atts ) {
2 // Shortcode functionality here
3}
4add_shortcode( 'custom_shortcode', 'custom_shortcode_function' );
Step 2: Implement the Shortcode Callback Function
Inside the callback function, you can define the functionality of your shortcode. This can range from simple content output to complex dynamic functionality.
1function custom_shortcode_function( $atts ) {
2 // Extract shortcode attributes
3 $atts = shortcode_atts( array(
4 'param1' => 'default_value',
5 'param2' => 'default_value'
6 ), $atts );
7
8 // Process shortcode attributes
9 $param1_value = $atts['param1'];
10 $param2_value = $atts['param2'];
11
12 // Shortcode functionality here
13
14 // Return output
15 return $output;
16}
Step 3: Embed the Shortcode in Theme Files
Once the shortcode is registered and the callback function is implemented, you can embed the shortcode directly into your theme's PHP files using the do_shortcode()
function.
1<?php echo do_shortcode('[custom_shortcode param1="value1" param2="value2"]'); ?>
Best Practices for Using Shortcodes in Theme PHP
When integrating shortcodes into your theme's PHP files, keep the following best practices in mind:
- Separation of Concerns: Try to keep your shortcode functionality separated from your theme's presentation logic for easier maintenance and scalability.
- Sanitization and Validation: Always sanitize and validate user input to prevent security vulnerabilities and ensure data integrity.
- Documentation: Document your custom shortcodes thoroughly to make it easier for yourself and others to understand their purpose and usage.
Conclusion
Integrating WordPress shortcodes into your theme's PHP files can greatly enhance the functionality and customization options of your website. By following the steps outlined in this guide and adhering to best practices, you can harness the power of shortcodes to create dynamic and engaging user experiences across your site.
Related
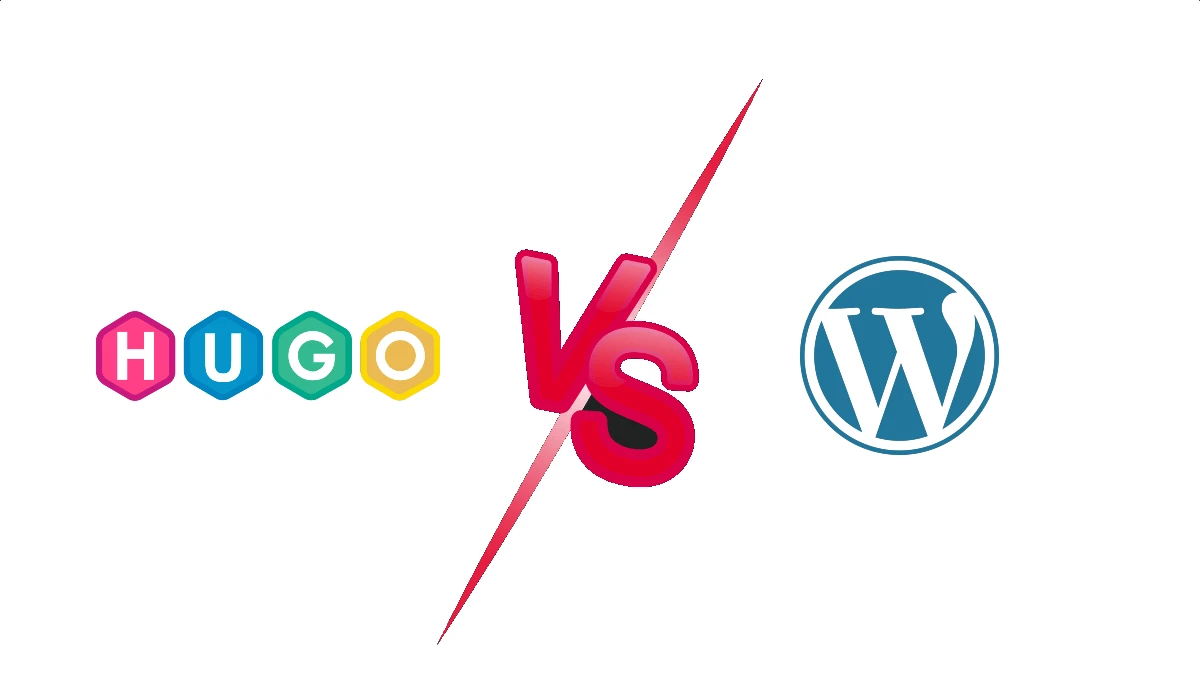
Choosing the Right Platform: Hugo vs. WordPress for Your Website
As technology evolves, developers and content creators are presented with an array of options. Among these, Hugo and WordPress stand out as robust solutions, each with its strengths and weaknesses. In this comprehensive exploration, we'll delve into various aspects to help you make an informed decision for your website. 1. Performance and Speed: When it comes to performance, Hugo and WordPress take divergent paths. Hugo, a static site generator, excels in speed due to its pre-rendered content.
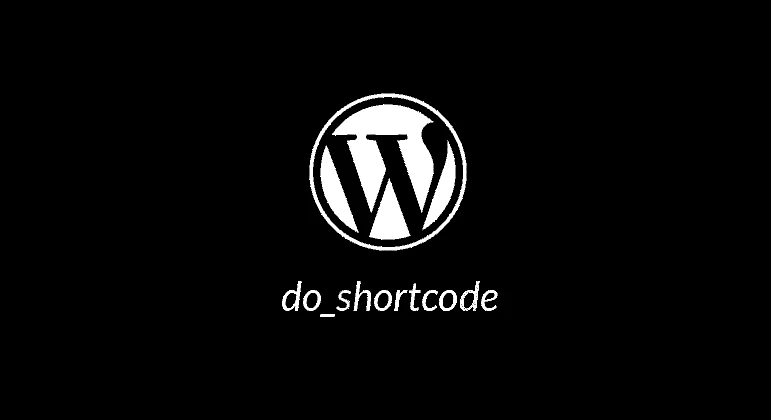
Harnessing the Power of WordPress Shortcodes in Your Theme's PHP
WordPress shortcodes are powerful tools that allow you to add dynamic content and functionality to your website with minimal effort. While they are commonly used within post content or widgets, integrating shortcodes directly into your theme's PHP files can offer even more flexibility and control over your site's design and functionality. In this guide, we'll explore the ins and outs of using WordPress shortcodes in your theme's PHP files, complete with examples and best practices.