How to Open Folders in Visual Studio Code from File Manager and Close Sessions Automatically
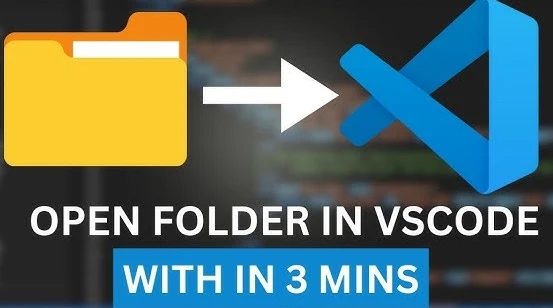
Overview
How to Open Folders in Visual Studio Code from File Manager and Close Sessions Automatically
Managing multiple projects and folders efficiently in Visual Studio Code (VS Code) can boost productivity, especially for developers. A common requirement is to open folders from the file manager into VS Code and ensure the folder or project closes automatically when VS Code is exited. This blog post covers several ways to achieve this on Windows, Linux, and macOS, including automation through scripts and customizing file manager actions.
If you're tired of leaving old project folders open in VS Code, or if you want to launch projects directly from your file manager with a clean experience, this guide will give you everything you need.
1. Prerequisites: Setting up VS Code CLI
Before we dive into scripting or automation, it’s important to ensure the VS Code command line interface (CLI) is set up properly.
Install the VS Code CLI (code
)
- Open VS Code.
- Press
Ctrl + Shift + P
(or Cmd + Shift + P on macOS) to open the command palette. - Search for and run:
Shell Command: Install 'code' command in PATH
This will allow you to use the code
command from your terminal or command prompt to open projects and folders in VS Code.
2. Opening a Folder with a Script
If you want to automate opening folders from your terminal or file manager and ensure they close properly when VS Code exits, follow these script-based solutions. These scripts will:
- Open a folder in VS Code.
- Monitor VS Code and exit the session once the app is closed.
2.1 Bash Script for Linux/macOS
Save the following as open-code.sh
:
1#!/bin/bash
2
3# Check if a folder path is provided
4if [ -z "$1" ]; then
5 echo "Usage: $0 <folder-path>"
6 exit 1
7fi
8
9# Open the folder in VS Code
10code "$1"
11
12# Wait for VS Code to close
13while pgrep -x "code" > /dev/null; do
14 sleep 1
15done
16
17echo "VS Code closed. Session ended."
Usage:
Save the script as
open-code.sh
.In your terminal, give it execute permissions:
1chmod +x open-code.sh
Run the script with the folder path:
1./open-code.sh /path/to/your/project
This script will wait for the VS Code process to exit and then print a message saying the session is ended.
2.2 Batch Script for Windows
Save the following as open-code.bat
:
1@echo off
2if "%1"=="" (
3 echo Usage: open-code.bat <folder-path>
4 exit /b 1
5)
6
7:: Open the folder in VS Code
8code "%1"
9
10:: Monitor VS Code until it's closed
11:loop
12tasklist | find /i "code.exe" >nul
13if not errorlevel 1 (
14 timeout /t 1 >nul
15 goto loop
16)
17
18echo VS Code closed. Session ended.
Usage:
Save the script as
open-code.bat
.Open the Command Prompt and run the script:
1open-code.bat "C:\path\to\your\project"
This script monitors the VS Code process and exits once the application closes.
3. Modify VS Code Settings to Manage Sessions
If you want each VS Code session to open fresh (without restoring previous folders or workspaces), you can modify the window settings.
Steps to Modify VS Code Session Behavior:
- Open VS Code and go to:
File > Preferences > Settings (or press
Ctrl + ,
). - Search for
window.restoreWindows
and set it tonone
.
This ensures that no previous session or folder opens when launching VS Code. - Optionally, search for
window.newWindow
and set it toon
.
This ensures every new folder opens in a separate instance of VS Code.
Alternatively, you can edit the settings.json
directly:
1{
2 "window.restoreWindows": "none",
3 "window.newWindow": "on"
4}
These settings ensure a clean, isolated session for each project.
4. Add Open with VS Code to File Manager
If you prefer to open folders directly from the file manager with VS Code, follow these platform-specific instructions.
4.1 Linux File Manager (Nautilus/Thunar)
Open Nautilus (or your file manager) and go to: Edit > Preferences > Configure Custom Actions.
Add a new action:
Name: Open with VS Code
Command:
1code %f
Under Appearance Conditions, set:
- File Pattern:
*
- Condition: Directories only
- File Pattern:
Now, when you right-click a folder, you’ll see the option to “Open with VS Code.”
4.2 Windows Context Menu
Open Registry Editor (
regedit
).Navigate to:
HKEY_CLASSES_ROOT\Directory\Background\shell
Create a new key called
Open with VS Code
.Inside that key, create another key called
command
.Set the Default value of
command
to:1"C:\Path\to\Code.exe" "%V"
After this, you can right-click in any folder and select “Open with VS Code” from the context menu.
5. Conclusion
With the methods described in this post, you now have several ways to:
- Open folders directly from the file manager or terminal into VS Code.
- Ensure sessions are isolated and closed properly when the VS Code window is exited.
- Customize your file manager and context menus for quick access to your projects.
Whether you prefer automated scripts or file manager customization, these solutions can help streamline your workflow. Enjoy your more organized development environment!
Comments