Unveiling the Power of Brute Force: Cracking SHA-256 Hashes with Python
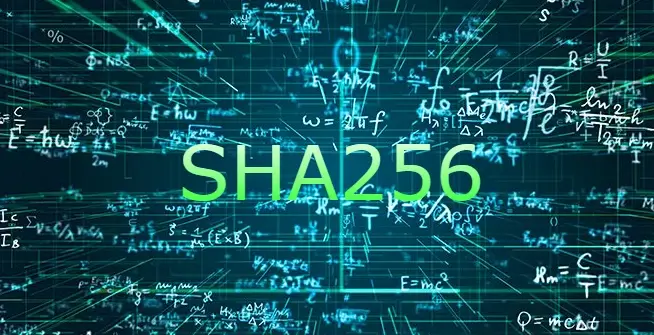
Overview
Hash functions, such as SHA-256, are integral to modern cryptography, ensuring data integrity and security. They are designed to be one-way functions—easy to compute but practically impossible to reverse. However, with enough computational power and persistence, brute force attacks can crack these hashes. In this blog post, we'll walk through a Python script designed to brute force a SHA-256 hash, breaking down each component to understand its functionality and the broader implications of such attacks.
Understanding the Script
The script provided demonstrates a brute force attack on a SHA-256 hash. Let's break down the script into manageable parts and understand its workings.
1. Importing Libraries
1import hashlib
2import random
3import string
We start by importing the necessary libraries:
hashlib
for generating SHA-256 hashes.random
andstring
for generating random passwords.
2. Generating a Unique Password
1def generate_unique_password(length, attempted_passwords):
2 characters = string.ascii_letters + string.digits
3 while True:
4 password = ''.join(random.choice(characters) for _ in range(length))
5 if password not in attempted_passwords:
6 attempted_passwords.add(password)
7 return password
This function generates a unique random password of a specified length. It ensures that each password is unique by checking against a set of previously attempted passwords.
3. Brute Force Function
1def brute_force_sha256(target_hash):
2 password_length = 64 # Length of the password
3 attempted_passwords = set()
4
5 while True:
6 guess = generate_unique_password(password_length, attempted_passwords)
7 hashed_guess = hashlib.sha256(guess.encode()).hexdigest()
8 if hashed_guess == target_hash:
9 return guess
10 print("Trying:", hashed_guess)
This function attempts to brute force the SHA-256 hash:
- It sets the password length to 64 characters.
- It continuously generates random passwords and checks their SHA-256 hash against the target hash.
- If a match is found, it returns the password.
4. Main Execution and Saving Results
1# Example usage
2target_hash = 'Your_Target_Hash_Script'
3result = brute_force_sha256(target_hash)
4
5# Save the cracked password to a file
6with open('cracked_password.txt', 'w') as file:
7 file.write(result)
8
9print("Decrypted value:", result)
10print("Cracked password saved to 'cracked_password.txt'")
In the main section of the script:
- A target hash is defined.
- The
brute_force_sha256
function is called with this target hash. - The resulting password is saved to a file and printed.
Implications and Considerations
While the script demonstrates the theoretical possibility of brute-forcing SHA-256 hashes, it's important to consider the practical implications:
- Computational Cost: Brute forcing is extremely resource-intensive and time-consuming. The script's effectiveness depends heavily on computational power and the length/complexity of the password.
- Security Measures: Modern security systems employ various measures to thwart brute force attacks, including rate limiting, account lockouts, and using salt with hashes.
Full Code
1import hashlib
2import random
3import string
4
5def generate_unique_password(length, attempted_passwords):
6 characters = string.ascii_letters + string.digits
7 while True:
8 password = ''.join(random.choice(characters) for _ in range(length))
9 if password not in attempted_passwords:
10 attempted_passwords.add(password)
11 return password
12
13def brute_force_sha256(target_hash):
14 password_length = 64 # Length of the password
15 attempted_passwords = set()
16
17 while True:
18 # Generate a unique random password
19 guess = generate_unique_password(password_length, attempted_passwords)
20 # Calculate the SHA-256 hash of the guess
21 hashed_guess = hashlib.sha256(guess.encode()).hexdigest()
22 # Check if the hashed guess matches the target hash
23 if hashed_guess == target_hash:
24 return guess
25 # Print the currently attempted hash
26 print("Trying:", hashed_guess)
27
28# Example usage
29target_hash = '06629dd1911f885409686333d5aaf58953a4d156d44b2447071ae3ed519867d6'
30result = brute_force_sha256(target_hash)
31
32# Save the cracked password to a file
33with open('cracked_password.txt', 'w') as file:
34 file.write(result)
35
36print("Decrypted value:", result)
37print("Cracked password saved to 'cracked_password.txt'")
Conclusion
This exploration of brute force attacks against SHA-256 hashes in Python sheds light on the balance between computational power and cryptographic security. While brute force remains a theoretical threat, practical implementations require significant resources and time. Strengthening security measures and staying informed about potential vulnerabilities are crucial steps in maintaining robust cybersecurity.
Feel free to try the script and experiment with different hash values, but always remember to use such knowledge responsibly and ethically. Cybersecurity is a continuous battle, and understanding the tools at our disposal is key to staying ahead.
Comments